Table of Contents
- C# Interview Questions and Answers
- What is C#? Explain its features.
- What are the differences between classes and objects in C#?
- Describe in detail the various C# classes.
- How do you go into great depth on object-oriented concepts?
- Can you explain how C# code is compiled?
- Could you elaborate on the use of ‘using’ statements in C#?
- Describe the method’s C# disposal in detail.
- Can you explain the finalized method in C# in detail?
- In C#How does one handle the passing argument differently?
- Key Takeaways
- FAQs
C# Interview Questions and Answers
C#, which emerged in 2000, has grown to become one of the major programming languages. The language, being a multi-paradigm programming language, also offers several functional programming capabilities that extend its utility and adaptability. Since C# is such a popular programming language, many large and small businesses base their products on it. So, to ace, prepare basic and advanced level C# interview questions.
What is C#? Explain its features.
C# is an object-oriented programming language created by Microsoft in the year 2000. Different operating systems are compatible with it. Additionally, C# is a general-purpose programming language used to build different programs and applications. We can use it to not only build Windows UI apps, backend services, controls, libraries, Android apps, but even blockchain apps. C#, like Java, is based on the notion of classes and objects.
The following are some of the C# features:
- Methodical approach.
- Simple to pass parameters.
- Code compilation is on different platforms.
- Open-source.
- Object-oriented.
- Adaptable and scalable
What are the differences between classes and objects in C#?
C# is an object-oriented programming language with classes as its base. A class is a representation of data structure, storage, and management inside a program. A class defines by its attributes, methods, and other objects.
Objects are real-world entities with specific properties that produce using class instances. The type of object determines these classes. The class is a Book, and it contains two properties: name and author.
Describe in detail the various C# classes.
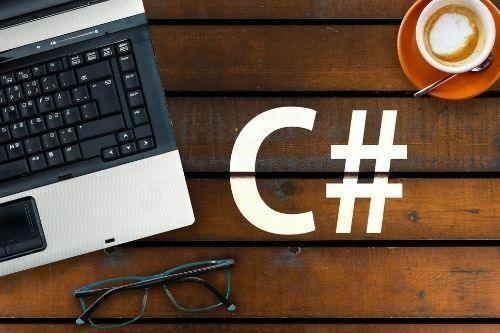
In C#, there are four different types of classes that we can use-
- Static Class: This is a type of class that cannot be instantiated in other words, we can’t construct an object of that class with the new keyword, and class members can only be accessed by using their class name.
- Abstract Class: The abstract keyword is used to declare abstract classes. However, for abstract classes, objects cannot be produced. It must inherit in a subclass if you wish to use it. Within an Abstract class, you can simply define abstract and non-abstract methods. The methods in the abstract class can either have or not have an implementation.
- Partial Class: This is a class that allows you to divide your properties, methods, and events into various source files, which then merge into a single class at build time.
- Sealed Class: A sealed class can’t be inherited from another class, and its attributes are limited. The sealed class is unaffected by any access modifiers.
How do you go into great depth on object-oriented concepts?
The object-oriented programming language C# supports four OOP principles.
Encapsulation: This is a term that refers to the process of tying together code and data and keeping it secure from other programs and classes. It’s a container that blocks code and data access by a program specified outside of it.
Abstraction: To promote efficiency and security inside the program, this object-oriented notion shields anything other than the relevant data about any produced object.
Inheritance: The use of inheritance is when one item makes use of the properties of another object.
Polymorphism: It’s a feature that lets one interface serve as a foundation class for additional interfaces. The phrase “one interface, many actions” frequently describes this notion.
Can you explain how C# code is compiled?
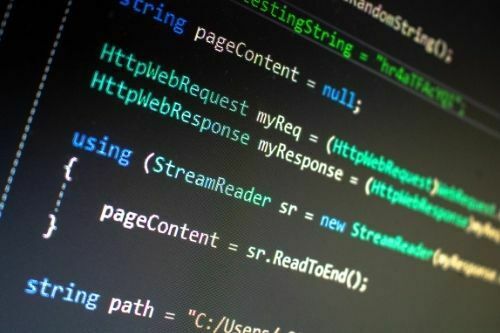
There are four phases to compiling code in C#. The steps are as follows:
1st step – compile the source code in the managed code that is compatible with the C# compiler.
2nd step – put the freshly developed code together into assemblies.
3rd step – Load the CLR (Common Language Runtime).
4th step – Use CLR to run the assembly and create the result.
Could you elaborate on the use of ‘using’ statements in C#?
The using statement sets one or more than one resource. Resources use and release constantly. This statement’s main purpose is to manage and release unneeded resources automatically. When you’re creating an object that’s utilizing the resource, be sure to use the object’s disposal method to release the resources it’s consuming.
Describe the method’s C# disposal in detail.
Dispose of the method by A class object’s unused resources are released by the disposeof() function. Unused resources such as files, data connections, and so forth. This function is defined in the IDisposable interface, which is implemented by the class by defining the IDisposable body interface. However, the disposal method is not invoked automatically; the programmer must explicitly implement it for effective resource usage.
Can you explain the finalized method in C# in detail?
The finalize () function is defined in the object class that is used for cleanup operations. The garbage collector usually calls this function once an object’s reference isn’t utilized for a long period. Garbage collector frees managed resources automatically, but you must explicitly implement the finalize function to free unneeded resources like filehandles, data connections, and so on.
In C#How does one handle the passing argument differently?
Any defined method can be given parameters in one of three ways, such as-
Value parameters: The real value of the parameter will be sent to the formal parameter. In addition, any modifications made to the formal parameter of the function will have no influence on the actual value of the argument in this circumstance.
Reference parameters: You may use this technique to transfer an argument that refers to a memory location into a formal parameter, which implies that any changes to the parameter will affect the argument.
Output Parameters: This method returns many values to the procedure.
Key Takeaways
- C# is a simple, contemporary, and general-purpose Object-oriented programming language included in Microsoft’s .NET framework.
- C# is becoming increasingly popular, and it now plays a significant part in the software testing industry.
We hope this blog was informative. Click here to reach out to us for more information on the Top C# interview questions and answers for 2022. We would be happy to assist you with your queries!
Liked this blog? Read next: Top 15 HTML interview questions & answers!
FAQs
Q1. In C# interview questions, what are generics?
Answer – Generics is a mechanism for writing class code without identifying the data type(s) on which the class operates. It also enables you to construct type-safe data structures without having to commit to specific data types.
Q2. In C# Interview Questions, what is abstraction?
Answer – An abstract method is a method that does not have a body. In order to declare it, we use an abstract modifier. It contains the method’s declaration/signature and not its implementation/body. Subsequently, to end an abstract function, we use a semicolon.
Q3. In C# Interview Questions, what is namespace?
Answer – The namespace keyword declares a scope that contains a group of linked items. A namespace can be used to structure the software.