Table of Contents
Both the structure and the union are user-defined data types in C Language. While they are fundamentally the same, they vary slightly in some ways, such as how memory is allocated to their members. Structure and union declare both in a similar way but perform differently. They enable the user to group different data types under a single name. When declaring structure variables, each member has its memory location; however, the various members of a union variable share the exact memory location. Let’s look at the difference between structure and union in greater depth.
History of the C language
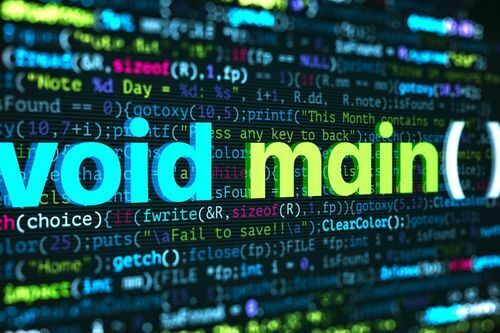
Dennis Ritchie created C, a successor to the programming language B, at Bell Labs between 1972 and 1973 to build utilities that ran on Unix. They used it to re-implement the Unix operating system’s kernel.
C gradually gained popularity during the 1980s. C compilers are available for almost all modern computer architectures and operating systems, making them one of the most widely used programming languages. The International Organization for Standardization (ISO) and American National Standards Institute (ANSI) have both standardized C since 1989.
What is a structure?
A structure is a collection of elements of the same data type. It is a programming language in C that enables users to combine logically related data items. Structures are essentially representations of records. Its elements are all stored in adjacent memory locations. A structure type, variables, can store multiple data items under the name.
The keyword ‘struct’ defines a structure containing multiple data types under a single name. For example, if you want to create an employee database, you must store the employee’s name, age, phone number, and salary information in a single entity. You can accomplice this through the keyword struct, which informs the compiler that a structure has been asserted.
struct employee
name;
age;
phone;
salary;
} emp1, emp2;
In this case, the type of the structure is called ‘employee,’ and two variables named ‘emp1’ and ‘emp2’ are created. There must be a semicolon after the closing braces (;).
There are two categories of operators that can access members of a structure-
- Member operator
- Structure pointer operator
What is a union?
A union is a user-defined type of data. It’s the same as the structure. The keyword for union is ‘union.’ The union combines various objects of different types and sizes. A user can define a union with multiple members, but only one of them is active at any given time. It allows you to use a single memory location for various purposes.
Unions only allocate the memory needed to hold the union’s largest member and allow the other data items to share the same memory location.
Since the address is the same for all members of a union, each member begins at the same offset value. Changing the value of one member has an effect on the values of the other members. When you want to store something that is one of several data types, you can use a union.
union employee {
char name [32];
int age;
float salary;
};
In this instance, the keyword ‘union’ defines the union, which is very similar to the structure declaration. The variable can hold a string value representing the employee’s name, a numeric value representing the employee’s age, or a float representing the employee’s salary.
Difference between structure and union in tabular form
Structure | Union |
The keyword ‘struct’ is used to define a structure. | The keyword ‘union’ is used to define a union. |
A structure’s members do not share memory. | A union’s members share memory space. |
You can retrieve any member at any time in a structure. | In a union, you can access only one member at a time. |
A structure’s members can all be initialized at the same time. | You can initialize only the first member. |
The structure’s size is equal to the sum of the sizes of its members. | The union is the same size as its largest member. |
Changing the value of one member does not affect the value of another. | A change in one member’s values will affect the other members’ values. |
Stores various values for all members. | Stores the same value for all members. |
Which one is better, structure or union?
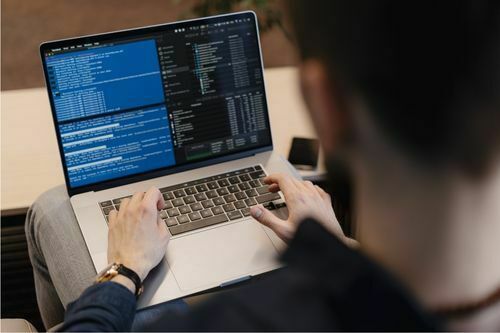
When working with multiple data types, structures are better suited for dependable and consistent performance. On the other hand, unions require less memory and are more effective when dealing with many members or objects.
Structures are convenient, but they are not designed for large projects. Differences in structure and union in C language are easily noticeable when working with different data types with different attributes or when there are many data items.
Key takeaways
- Structure and union are user-defined data types in the C programming language. While they are profoundly identical, they differ in some ways.
- Member operator and structure pointer operator are the operators that can access the member of a structure.
- Structure and union can differ through keywords, size, memory, value, and more.
Did you find this blog informative? If so, please share your thoughts in the comments below. Click here to contact us for more information on the difference between structure and union. We would be happy to assist you with your queries.
Liked this blog? Read next: C++ interview questions for experienced | Top questions for professionals
FAQs
Q1. Which is better, structure or union?
Ans- Union is the best option if you want to use the same memory location for two or more members.
Q2. What is the difference between structure and array?
Ans-Structure is a data structure that serves as a container for variables of various types. Array, on the other hand, is a type of data structure used as a container for variables of the same type.
Q3. What are pointers in C?
Ans-A pointer is a variable that stores the memory address of another variable as its value.