Table of Contents
- Why was Angular developed?
- What Is the Primary language used in Angular?
- What are the differences between Angular 1.x and 2.x?
- Explain Bootstrapping in Angular
- What is the difference between Promises and Observables?
- What are Ng-content and ContentChild?
- Difference between Component and Directive?
- What is a structural directive?
- How to dynamically delete components in Angular?
- What is a constructor? Why do we use it?
- Key Takeaways
- FAQs
Angular is one of the most in-demand skills in today’s employment market, but it’s also incredibly tough to grasp the complete framework, especially during an interview. It’s also very broad and tough to navigate. Even more challenging is answering Angular interview questions because it is tough to identify which aspects of the Angular framework you should be familiar with.
Keeping this in mind, we’ve compiled a list of the popular Angular interview questions to help you ace your interviews.
Why was Angular developed?
Angular was designed to generate single-page applications. This framework gives online applications structure and consistency while also delivering great scalability and maintainability. It is a TypeScript-based open-source JavaScript framework. It employs HTML syntax to define the components of your application clearly.
What Is the Primary language used in Angular?
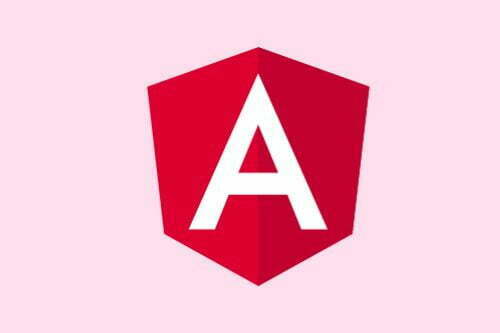
Angular is based on TypeScript and HTML. HTML is used for the template, and TypeScript (a superset of JavaScript) is used for components.
What are the differences between Angular 1.x and 2.x?
The latest version of Angular is 7.x. This means that it is a complete rewrite of Angular 2.x, which was released in 2016. Let’s compare the two:
- Angular 7 is a major release that happens every three months or so (like clockwork), whereas Angular 4 was released after a year and a half, which means it’s not as stable as the previous versions for your project requirements (yeah I know, but sometimes it’s just true).
- There are no breaking changes between versions 3 and 4 of this framework; however, some minor improvements were made within those two releases that could have affected some small parts of your codebase if they were written with backward compatibility in mind (like using TypeScript instead). For example: If you had used any deprecated API function from previous versions, then it might stop working when upgrading to newer ones because its functionality has been replaced by something else entirely different now!
Explain Bootstrapping in Angular
Bootstrapping is the process of loading the Angular framework into your application. This allows you to use Angular’s built-in directives and components. In a simple case, bootstrapping usually means creating an instance of the `ngApp` directive and adding it to your root HTML element. This tells Angular that this is where it should start its work.
What is the difference between Promises and Observables?
The main difference between promises and observables is that the former emit a single value, while the latter can emit multiple values over time.
You should use promises when you are making synchronous calls to your API, such as when fetching something from the server or using jQuery’s $.ajax(). Observables are better suited for asynchronous calls or other situations where additional values may be emitted at some point in time.
Another key difference is that callbacks are used with promises, while we have async/await syntax as well as flatMap()/switchMap() operators available with observables. These let us handle success and failure cases better by providing an opportunity to react intelligently based on what happens inside our code (e.g., if there was an error).
Additionally, both promise resolution and subscription cancellation happen outside of any observable processing; whereas with observables, we need to define what happens when either occurs inside the handlers.
What are Ng-content and ContentChild?
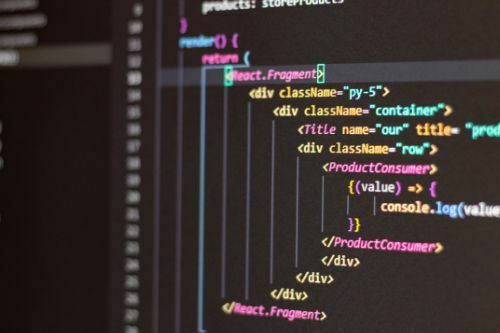
You are going to be asked about NgContent and ContentChild. You can use these two directives inside a component to render multiple components and pass data from one component to another. NgContent is used for rendering a single child component into the template of another component.
Difference between Component and Directive?
The difference between a component and a directive is that a component defines a new HTML element, whereas a directive modifies existing HTML elements.
A component can be thought of as a class, while a directive is just an interface with some functionality defined in it.
You can also think of a component as a decorator because it wraps around an existing HTML tag (or element).
What is a structural directive?
A structural directive is a way to create and organize components in Angular. It allows you to add custom elements that can be used throughout your templates.
When structural directives are applied they generally are prefixed by an asterisk, *, such as *ngIf. This convention is shorthand that Angular interprets and converts into a longer form. Angular transforms the asterisk in front of a structural directive into an <ng-template> that surrounds the host element and its descendants.
How to dynamically delete components in Angular?
You can use the ngOnDestroy() method to destroy your components. This method is called when a component is destroyed and removed from the DOM. It gets invoked before its siblings are destroyed, except for the host element’s children, which are destroyed after the host element itself.
What is a constructor? Why do we use it?
A constructor is a special type of function that’s called when you create a new instance of your class. The constructor initializes the class and its members, so it’s often used to set up the state of an object right after it has been created.
Key Takeaways
- Angular is a complex platform that’s difficult to learn, so it requires proper qualifications and preparation from a developer.
- The diversity of Angular capabilities, for instance, template syntax, Angular CLI, routers, etc. make programmer’s work easier and enable quick loading of the application.
- Angular has a few imperfections related to the development process. However, the framework evolves rapidly, and its community offers various alternatives and solutions to problems.
Liked this blog? Read next: Top 15 HTML interview questions & answers!
FAQs
Q1. What should I do to prepare for the Angular interview?
Answer – The following pointers will assist you in preparing for your Angular interview: Maintain your knowledge of several programming languages, such as JavaScript, TypeScript, and HTML coding, because working with Angular may need the use of distinct language applications.
Q2. Is Angular a viable career path?
Answer – You will be able to collaborate with a variety of Angular-related organizations. Because Angular is so popular among big software firms, being an Angular-equipped software developer is a lucrative, growing, and intriguing career option with a very bright future.
Q3. What is a decorator in Angular interview questions?
Answer – When designing an angular framework with version 2 and higher, the essential concept is a decorator. It could soon become a standard part of JavaScript. Decorators are widely utilized in Angular 4, and they are also used to build code.