Table of Contents
- What is polymorphism? Does it support C++?
- What is a real-life example of polymorphism?
- What is structural programming?
- What is encapsulation?
- What are the limitations of inheritance?
- What is abstraction?
- How is an abstract class different from an interface?
- Explain the advantages of abstraction?
- What are tokens?
- What is a constructor?
- What is the difference between a structure and a class?
- What are the different types of access modifiers?
- What we have to say
- FAQ’s
The OOPs model uses popular languages like Java, C++, and Python, among others, and is becoming more important in the programming industry. A user may utilize OOPs to break down their software into manageable chunks that solve quickly, such as one object at a time. In addition, the system that uses the OOP paradigm can quickly update, resulting in higher software quality, increased programmer productivity, and cheaper maintenance costs. The interview process for OOPs may include an array of questions. Check out the most frequently asked OOPs interview questions below!
What is polymorphism? Does it support C++?
Polymorphism describes the behavior of certain codes, processes, or objects in diverse situations. Polymorphism supports C++ through the following features –
- Build Time Polymorphism- When a polymorphic call is made, the compiler knows which function should be called at compile time. Templates, method overloading, and default parameters are all characteristics that facilitate compiler time polymorphism in C++.
- Run-Time Polymorphism- Virtual functions allow run-time polymorphism. The concept is that virtual functions are named after the kind of object pointed to or referenced, rather than the type of pointer or reference. To put it another way, virtual functions resolve at runtime.
What is a real-life example of polymorphism?
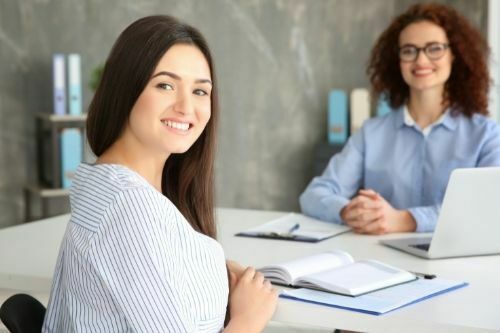
The first example is using virtual functions in C to output a list of objects that tie up with multiple functions to deliver different outcomes. This, along with the fact that the behavior of the items on the list isn’t available until runtime, is a fantastic real-world illustration of polymorphism.
What is structural programming?
The classic approach to programming, which revolves around functions, is rightly structural programming. In order to give a logical framework, functions separate the total program logic. It follows a top-down strategy. Structural programming is appropriate for issues that are simple to moderately difficult.
What is encapsulation?
Encapsulation is a property of an object that contains all concealed data. The concealed data is restricted only to the members of the class.
The different levels of encapsulation include public, protected, private, internal, and protected internal.
What are the limitations of inheritance?
Yet another most important OOPs interview question is about inheritance. Here’s how you should answer this question.
Inheritance needs additional processing time because the programs must browse numerous classes during execution. The parent and child classes inextricably link due to inheritance. Any modifications to the logic may necessitate updates to both the parent and child classes.
If there is a problem in implementing inheritance, it might result in undesirable outcomes.
What is abstraction?
You don’t want to know how the software’s components to function or how it creates if you’re a user with a problem statement. You just care about how the program resolves your issue. Abstraction is a technique for separating important features from those that aren’t. It is one of the most important characteristics of OOPs.
How is an abstract class different from an interface?
The OOPs interview questions can get into further detail with the above question. Here’s how you should answer the question.
Both an interface and an abstract class are special sorts of classes that only include the specification of methods and not their implementation. However, the interface is not the same as an abstract class. The key distinction between the two is that when an interface implements, the subclass is responsible for defining all the interface’s methods and implementing them. When an abstract class is inherited, the subclass is not required to define its abstract method until the subclass uses it.
Explain the advantages of abstraction?
Abstraction shows the user only the most important facts while ignoring or hiding extraneous or complicated features. To put it another way, data abstraction reveals the interface while concealing implementation specifics. Java uses interfaces and abstract classes to accomplish abstraction. The benefit of abstraction is that it makes things easier to understand by eliminating or hiding implementation complexity.
Code duplication reduces, and reusability improves. The user is only shown important information, which increases the application’s security.
What are tokens?
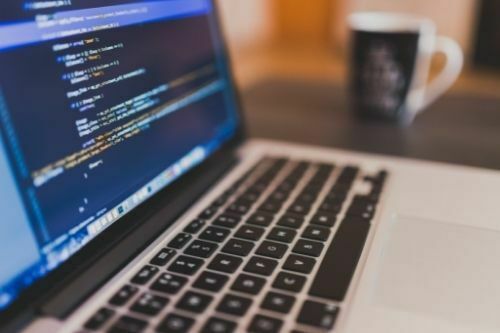
A token’s identity goes by a compiler and cannot be broken down into its constituent components. Tokens include keywords, identifiers, constants, string literals, and operators.
Punctuation characters are also tokens. Brackets, commas, braces, and parentheses are some examples of tokens.
What is a constructor?
In object oriented programming, a constructor is a sort of subroutine that uses to build a project. It seems like an instance method, but it doesn’t have a return type.
Constructors vary from conventional functions in the following ways:
- There is no return type for constructors.
- The constructor takes the name as the class.
- You call the constructor when you create an object.
What is the difference between a structure and a class?
The OOPs interview questions can also comprise questions of differentiation, such as this. Here’s how you should answer the question.
A Structure’s default access type is public, whereas a class’s access type is private. A structure organizes data, whereas a class organizes data and methods. Structures are used just for data and do not require stringent validation. However, classes encapsulate inherent data and require strict validation.
What are the different types of access modifiers?
Access modifiers support OOPs in the following four different ways-
- This modification is accessible from any location. Within the package, outside the package, within, as well as outside the class are the access levels for this modification.
- Outside of the class, you can use this modification. However, the access level is limited to the class.
- This modifier’s access level is protected within the package, through the child class, and outside the package. You can’t access child class from outside the package if you don’t specify it.
- Default: You can only access this modification within the package, and you can’t access it outside of it. If no access level is given, the default will be used.
What we have to say
- Nowadays, IT giants need and hire people who are knowledgeable about object-oriented techniques and patterns and are regularly conducting interviews for these positions.
- The benefit of recruiting such personnel is that they may quickly learn other OOP languages as needed by the company.
- You can boost your chances of being hired by organizations if you are properly prepared for OOPs interview questions.
Liked the blog? Comment below and share your thoughts with us!
Liked this blog? Read next: Computer Science Engineering Subjects | All you need to know
FAQ’s
Q1. What are the 3 basics of OOP?
Answer – The 3 basics of OOP include –
- Abstraction
- Encapsulation
- Inheritance
- Polymorphism
Q2. Is Python an OOP?
Answer – Yes, Python is an OOP. Excluding control flow, everything in Python is an object.
Q3. Why are OOPs important in the industry?
Answer – It aids in the writing of cleaner code and the control of functions and modules.