Table of Contents
What is the Spring Framework?
The Spring framework is an open-source framework designed to develop and support lightweight enterprise web applications. Also known as Spring, the framework can be seen as a collection of other Java (sub) frameworks. Including Spring AOP, Spring Web MVC, Spring DAO, Spring Context, Spring ORM, Spring Core, and Spring Web Flow. Enterprises can develop robust, scalable Java applications using these seven loosely-coupled modules.
How does it work?
Each Spring module is designed to perform specific tasks. Like the Core, which specifies the dependencies within the business logic and decouples configurations. Or the DAO module, which provides data access for the application.
However, these seven modules can be clubbed in a way that creates three layers within the Spring framework-
- Presentation layer. Responsible for user interaction.
- Data access layer. Deals with data storage and retrievals.
- Logic layer. Contains the logic of the program.
These layers interact with each other, and yet, are designed to offer a decoupled architecture, ensuring that changes in one module do not affect the others.
Core logic
Spring is widely used owing to its distinct features for developing simple, reliable, and scalable enterprise applications-Dependency Injection and Inversion of Control.
- Dependency Injection. Dependency Injection is a coding technique that ensures two objects are loosely coupled; that changes in one do not affect the other. One way to do this, for example, is by using post-construction setter methods.
- Inversion of Control (IoC). At the core of the Spring Framework, IoC assigns the responsibility of instantiating, configuring, and assembling dependencies between objects. The IoC container, part of the Spring Core module, is responsible for this.
Spring MVC Architecture
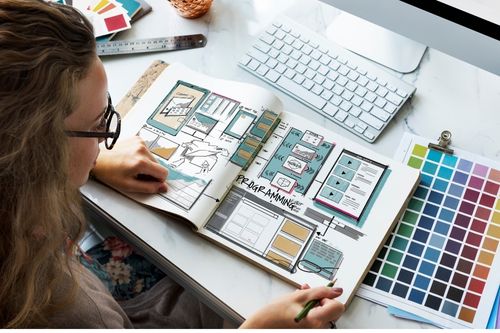
The Spring Web MVC is a module within the Spring Framework specifically designed to create lightweight and flexible web applications. It uses the Model-View-Controler pattern to implement the two core Spring features mentioned above-Dependency Injection and Inversion of Control.
How does it work?
The Spring MVC Architecture works around DispatcherServlet. DispatcherServlet is a class that receives incoming web requests, maps them to the right sources, and returns the responses. It does so by interacting with the three components within the Spring MVC Architecture-
- Model. It contains the application data.
- View. The view renders the data in the model and provides an HTML output to the Dispatcher Servlet.
- Control. This module is responsible for handling the requests made by the Dispatcher Servlet and providing it with the required Model and View.
The Spring MVC Framework functions like this
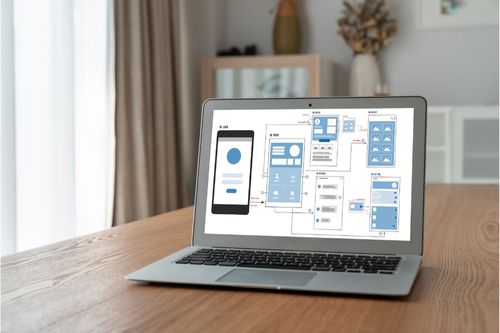
- The DispatcherServlet dispatches requests to handlers with configurable mappings and view, locale, and theme resolutions.
- The handler responds to the DispatcherServlet with the Controler name it should forward the request to.
- The controller returns a ModelAndView object to the DispatcherServlet.
- The DispatcherServlet invokes the relevant view component and gets a response for the request.
Environment set up
Follow these steps to set up the environment for your Spring application-
- Install the latest version of JDK (Java Development Kit) from the official Oracle website.
- Get the latest version of Apache Commons Logging API.
- Set up Eclipse IDE. You can also use IntelliJ to write the code.
- Download the Spring Framework Libraries.
If you get stuck at any step, do a simple Googe search and follow the instructions provided in the example videos.
Spring MVC Architecture example
1. Load the Spring jar files
Add the dependencies in a file titled pom.xml. You can also use Maven to do this.
- Spring Web MVC
- Spring Framework
- Servlet API
2. Define the Controller class
@Controller
public class Hello {
@RequestMapping(“/”)
public String display()
{
return “Hello”;
}
}
3. Create the web XML file
<?xml version=”1.0″ encoding=”UTF-8″?>
<web-app xmlns:xsi=”http://www.w3.org/2001/XMLSchema-instance”
xmlns=”http://java.sun.com/xml/ns/javaee”
xsi:schemaLocation=”http://java.sun.com/xml/ns/javaee
http://java.sun.com/xml/ns/javaee/web-app_3_0.xsd” id=”WebApp_ID” version=”3.0″>
<display-name>SpringMVC</display-name>
<servlet>
<servlet-name>spring</servlet-name>
<servlet-class>org.springframework.web.servlet.DispatcherServlet</servlet-class>
<load-on-startup>1</load-on-startup>
</servlet>
<servlet-mapping>
<servlet-name>spring</servlet-name>
<url-pattern>/</url-pattern>
</servlet-mapping>
</web-app>
4. Define the bean in another XML file
<?xml version=”1.0″ encoding=”UTF-8″?>
<beans xmlns=”http://www.springframework.org/schema/beans”
xmlns:xsi=”http://www.w3.org/2001/XMLSchema-instance”
xmlns:context=”http://www.springframework.org/schema/context”
xmlns:mvc=”http://www.springframework.org/schema/mvc”
xsi:schemaLocation=”
http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans.xsd
http://www.springframework.org/schema/context
http://www.springframework.org/schema/context/spring-context.xsd
http://www.springframework.org/schema/mvc
http://www.springframework.org/schema/mvc/spring-mvc.xsd”>
<context:component-scan base-package=”add package name” />
<mvc:annotation-driven/>
</beans>
5. Display the message using JSP
<html>
<body>
<p>Spring MVC Architecture Tutorial!!</p>
</body>
</html>
6. Start the server and run your project!
Key takeaways
- The original Java web applications were difficult to design and implement.
- The Spring Framework was introduced to help enterprises develop lightweight and flexible applications.
- It contains features that allow objects to interact with each other while being loosely coupled.
- The framework consists of seven sub-frameworks.
- Spring Web MVC is one of these sub-frameworks within the Spring architecture. It implements all the features of the Spring framework.
- The Spring MVC architecture uses a DispatcherServlet to handle web requests.
- Follow the steps given in this blog to write your own Spring web application.
Like this blog? Read next: Java Games | 8 Best books aspiring Java developers must know about!
FAQs
Q1. What do you mean by MVC architecture?
Ans – It is a program development architectural pattern designed to create applications using three distinct components-Model, View, and Controller.
Q2. Is MVC architecture a three-tier architecture?
Ans – No, it is a triangular architecture.
Q3. Can MVC architecture be used in Java?
Ans – Yes, the Spring MVC architecture allows Java developers to create simple and reliable web applications.