Table of Contents
- What is a stack?
- What is an array?
- What is a multidimensional array?
- What is a linked list data structure?
- What are the advantages of a linked list over an array?
- Why do we need an algorithm analysis?
- How does binary search work?
- What is a merge sort?
- What is the working of a selection sort?
- How does Huffman’s algorithm work?
- What does a deque mean?
- Key takeaways
- FAQ’s
Computers have been the key factor behind every discipline’s advancement and progression. With rapid developments in business and other industries, the ability to handle large amounts of data has become critical. To meet the need, new areas such as data analytics and data science have exploded in terms of employment. Students often have difficulty answering data structure interview questions when applying for their dream jobs. But if properly prepared, it can be an easy breeze to ace the interview round!
What is a stack?
A stack, also known as a Last-In-First-Out (LIFO) list, operates as an ordered list where insertion and deletion can only occur at one end, which is the top. It features a top element pointer and serves as a recursive data structure. In this structure, the element entered first in the stack is removed last.
What is an array?
Arrays keep collections of comparable sorts of data nearby in memory.
In the most basic data structure, one can retrieve a data element at random using its index number alone.
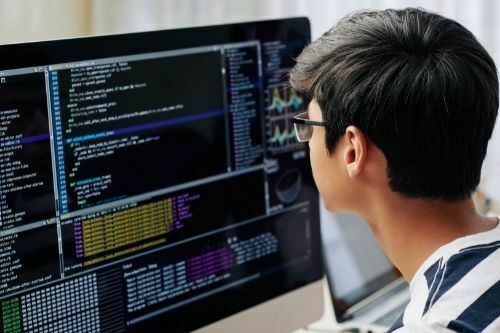
What is a multidimensional array?
A multidimensional array is an array that has more than one dimension. It’s either an array of arrays or an array with several levels. The two-dimensional array, or 2D array, is the simplest basic multidimensional array. It’s technically an array of arrays, as you’ll see in the code. A 2D array is also known as a matrix or a table with rows and columns. For a 2D l array, we must inform C that we have two dimensions.
What is a linked list data structure?
This is one of the most common data structure interview questions, and the interviewer wants you to provide a detailed response. Instead of concluding your answer in a phrase, try to describe as much as possible!
A linked list is a linear data structure where a succession of data objects is formed by connecting the elements with pointers. The elements in a linked list are not stored in the same memory address. Each element, known as a node, serves as a distinct entity. A data field and a reference to the next node are both present in each node. The head is the starting point of a linked list. The head is a null reference. The final node is also a null reference when the list is empty.
A linked list is a dynamic data structure with an unlimited number of nodes and the capacity to grow and shrink on demand.
What are the advantages of a linked list over an array?
- One can increase the size of a linked list at runtime, which is not possible with an array.
- The List does not need to be contiguous in the main memory. If contiguous space is not available, the nodes can be put anywhere in the memory and connected by links.
- The main memory dynamically stores the List, allowing it to expand according to program demands. On the other hand, the array is statically stored in the main memory and requires a predefined size during compilation.
- The available memory space determines the limit on the number of items that can be stored in a linked list. In contrast, the size defined for the array determines the maximum number of elements it can hold.
Why do we need an algorithm analysis?
Several solution algorithms can be used to tackle an issue. Algorithm analysis estimates the resources required by an algorithm to solve a certain computer task. The quantity of time and space needed to complete the task is also calculated with the help of algorithm analysis.
The time complexity of an algorithm measures how long an algorithm takes to run as a function of the length of the input. Whereas the space complexity of an algorithm is a measure of how much space or memory it takes to run as a function of the length of the input.
How does binary search work?
This is yet another important data structure interview question. Here’s how you should answer it.
When prioritizing efficiency, individuals perform a binary search by sorting data in either ascending or descending order. They start the search by discovering the center element in the array and dividing it into two halves based on whether the search value is greater than or less than the middle element. Knowledge of the arrangement sequence becomes crucial for finding the desired value.
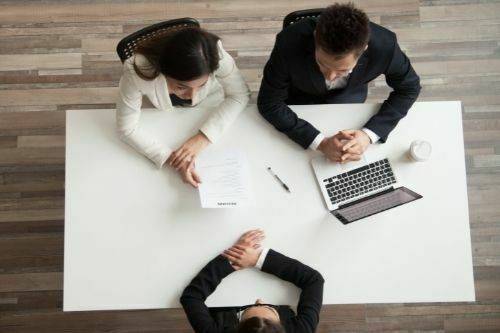
What is a merge sort?
Merge sorting is a sorting method based on the divide-and-conquer strategy. In every iteration, data entities that are next to each other are first merged and arranged to build sorted lists. In the end, these smaller sorted lists are linked together to make a fully sorted list.
What is the working of a selection sort?
In the realm of data structures, the selection sort stands out as a commonly employed sorting algorithm. The process follows a simple approach – it locates the smallest object and sets its index to zero, effectively placing it as the first element in the sorted sequence. The last stages entail iterating over the remaining items and adding the next index if needed. This process is repeated until all the items have been sorted.
How does Huffman’s algorithm work?
Huffman’s algorithm employs a table to store the frequency of occurrence for each data object in the list. This stored information is utilized to create extended binary trees, which are renowned for their ability to achieve the shortest path lengths. The algorithm takes into consideration all the appropriate weights during this process.
What does a deque mean?
Describing a deque also turns out to be a significant aspect of the data structure interview questions.
Deque s known as a double-ended queue. This means that any of the two ends can have items added or deleted. It may function as both a standard queue and a stack. In general, it outperforms both linked lists and stacks.
Key takeaways
- Present yourself by preparing well throughout your interview and taking the time to think about and arrange your responses beforehand.
- If you have any reservations about a question, rather than avoiding it, it is best to brush up on your knowledge of the subjects.
- Maybe you won’t be asked the exact same questions, but you can build on the information and learn more.
We hope you ace your interview and get your dream job! And if you have any problems or roadblocks, feel free to contact our experts!
Like this blog? Read next: Frequently asked interview questions | A guide to preparing well!
FAQ’s
Q1. What are the 4 data structures?
Answer – The 4 data structures are as follows –
- Array data structure
- Stack data structure
- Queue data structure
- Linked list data structure
Q2. Which language is the best for a data structure?
Answer – The best language for a data structure is –
- Python
- Java script
- Java
- C++
- C#
- Golang
- Swift
- PHP
Q3. What is a data structure in C?
Answer – The data structure in C is used to store data in an organized manner.